Dataset without labels. Which machine learning algorithm should you use?
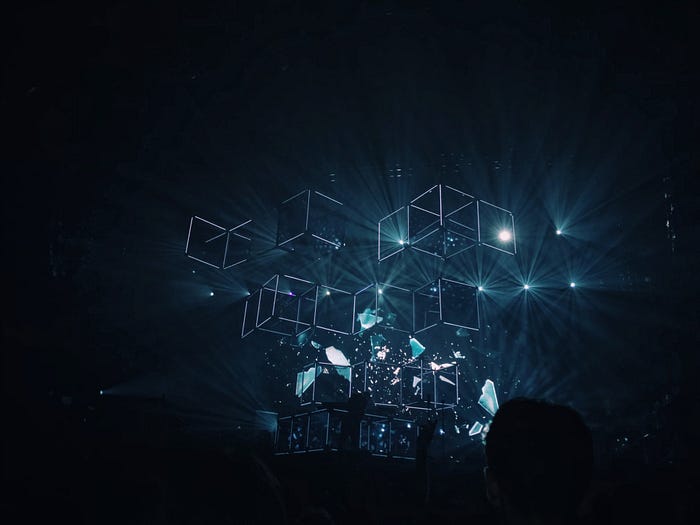
If you have a dataset without labels, you should use unsupervised learning algorithms. These algorithms are designed to work with data that has no predefined output or target variable. Instead, they aim to find patterns or relationships in the data itself.
Here are a few popular unsupervised learning algorithms that you can consider:
- K-means clustering: This algorithm groups data points into a predefined number of clusters (K) based on their similarity. The algorithm assigns each data point to the nearest cluster center (centroid), iteratively updates the centroids, and repeats the process until convergence. This method is useful for finding groups in your data. K-means clustering is a popular unsupervised learning algorithm used for partitioning a dataset into K distinct, non-overlapping clusters based on the similarity of the data points. The goal is to minimize the within-cluster sum of squares (WCSS), which is the sum of the squared distances between each data point and its corresponding cluster center (centroid).
Here’s a step-by-step breakdown of the K-means clustering algorithm:
- Initialize: Select the number of clusters, K, and randomly choose K initial cluster centroids from the dataset. These centroids can either be randomly chosen data points or computed by other initialization methods, such as k-means++.
- Assignment: Assign each data point to the nearest centroid based on a distance metric, such as Euclidean distance. The distance between each data point and all centroids is computed, and each data point is assigned to the centroid with the smallest distance.
- Update: Recalculate the centroids by taking the mean of all the data points assigned to each centroid. The new centroid positions represent the average of the data points in that cluster.
- Convergence check: If the centroids have not moved significantly or the maximum number of iterations has been reached, the algorithm stops. Otherwise, return to step 2 (Assignment) and repeat the process.
The K-means clustering algorithm has several advantages:
- It is easy to implement and understand.
- It is computationally efficient, especially for large datasets.
- It can be used as a preprocessing step for other machine learning algorithms.
However, it also has some limitations:
- The number of clusters, K, must be specified beforehand.
- The algorithm is sensitive to the initial placement of centroids and can get stuck in local minima. This issue can be mitigated by using techniques like k-means++ for initializing centroids or running the algorithm multiple times with different initializations and choosing the best result.
- It may not work well with clusters of different sizes, densities, or non-spherical shapes.
- It is susceptible to the “curse of dimensionality” when dealing with high-dimensional data. Dimensionality reduction techniques like PCA can be applied beforehand to alleviate this issue.
For a deeper understanding and see examples, you can check out the following resources:
- Scikit-learn’s K-means clustering documentation: https://scikit-learn.org/stable/modules/generated/sklearn.cluster.KMeans.html
- Coursera’s “Machine Learning” course by Andrew Ng (Week 8): https://www.coursera.org/learn/machine-learning
- StatQuest’s “K-means clustering” video tutorial: https://www.youtube.com/watch?v=4b5d3muPQmA
2. DBSCAN (Density-Based Spatial Clustering of Applications with Noise): DBSCAN (Density-Based Spatial Clustering of Applications with Noise) is an unsupervised learning algorithm that finds clusters based on the density of data points. Unlike K-means clustering, DBSCAN does not require specifying the number of clusters beforehand, and it can identify clusters of varying shapes and sizes, as well as noise points.
DBSCAN is a density-based clustering algorithm that can find clusters of different shapes and sizes, as well as identify noise points that do not belong to any cluster. It works by defining a neighborhood around each data point and connecting points that are close enough. Points that are not part of any cluster are treated as noise.
Here’s an overview of the DBSCAN algorithm:
- Parameters: Two key parameters must be defined: the radius (Eps) and the minimum number of points (MinPts) required to form a dense region.
- Core Points: A data point is considered a core point if there are at least MinPts number of points within the Eps radius, including the data point itself.
- Border Points: A data point is considered a border point if it has fewer than MinPts points within the Eps radius but is within the Eps radius of a core point.
- Noise Points: A data point is considered a noise point if it is neither a core point nor a border point.
- Clustering process:
- Start with an arbitrary core point that has not been visited.
- Create a new cluster for the core point and all the reachable data points within the Eps radius.
- Repeat the process for each unvisited core point.
- Noise points are not assigned to any cluster.
DBSCAN has several advantages:
- It can find clusters of different shapes and sizes.
- It can detect and separate noise points from the clusters.
- It does not require specifying the number of clusters.
However, DBSCAN also has some limitations:
- It is sensitive to the choice of Eps and MinPts parameters. Inappropriate values can lead to poor clustering results.
- It may not perform well with clusters of varying densities or when dealing with high-dimensional data. In the latter case, dimensionality reduction techniques like PCA can be applied beforehand to alleviate this issue.
- It has a higher time complexity compared to K-means, especially for large datasets.
To gain a deeper understanding and see examples, you can check out the following resources:
- Scikit-learn’s DBSCAN documentation: https://scikit-learn.org/stable/modules/generated/sklearn.cluster.DBSCAN.html
- DataCamp’s “Cluster Analysis in Python” course: https://www.datacamp.com/courses/cluster-analysis-in-python
- StatQuest’s “DBSCAN — Density-Based Spatial Clustering of Applications with Noise” video tutorial: https://www.youtube.com/watch?v=eq1zKgCFwkk
3. Hierarchical clustering: Hierarchical clustering creates a tree-like structure (dendrogram) of nested clusters, where each data point starts as its own cluster and is then combined with its closest neighbor to form a new cluster. This process is repeated until there is only one cluster left. You can choose to cut the dendrogram at a specific level to obtain the desired number of clusters.
Hierarchical clustering is an unsupervised learning algorithm that builds a hierarchy of clusters by successively merging or splitting data points based on their similarity or distance. There are two main approaches to hierarchical clustering: agglomerative (bottom-up) and divisive (top-down). The more commonly used approach is agglomerative hierarchical clustering, so I will focus on that.
Agglomerative hierarchical clustering works as follows:
- Initialization: Each data point starts as an individual cluster. So, if there are N data points, there will initially be N clusters.
- Compute distances: Calculate the distances between all pairs of clusters using a distance metric (e.g., Euclidean distance) and a linkage criterion (e.g., single, complete, average, or Ward’s linkage).
- Merge clusters: Find the pair of clusters with the smallest distance according to the linkage criterion and merge them into a new cluster. Update the distance matrix to reflect the new cluster.
- Repeat steps 2 and 3: Continue computing distances and merging clusters until all data points belong to a single cluster.
- Dendrogram: A dendrogram is a tree-like diagram that visually represents the hierarchical clustering process. Each merge is represented as a node in the dendrogram, and the height of the node corresponds to the distance between the merged clusters.
- Cut the dendrogram: To obtain the desired number of clusters, you can cut the dendrogram at a specific height (distance threshold). All clusters connected by a node below the chosen height will form separate clusters.
Hierarchical clustering has several advantages:
- It provides a hierarchical representation of the data, which can be useful for understanding the relationships between clusters.
- It does not require specifying the number of clusters beforehand. The desired number of clusters can be determined by cutting the dendrogram at an appropriate height.
- It can produce clusters with varying shapes and sizes.
However, hierarchical clustering also has some limitations:
- It is computationally expensive, especially for large datasets, as it requires computing the distance matrix for all pairs of clusters.
- It is sensitive to the choice of distance metric and linkage criterion, which can affect the quality of the clustering.
- Once clusters are merged or split, the decisions cannot be undone, which may lead to suboptimal clustering results.
To gain a deeper understanding and see examples, you can check out the following resources:
- Scikit-learn’s hierarchical clustering documentation: https://scikit-learn.org/stable/modules/clustering.html#hierarchical-clustering
- DataCamp’s “Cluster Analysis in Python” course: https://www.datacamp.com/courses/cluster-analysis-in-python
- StatQuest’s “Hierarchical Clustering” video tutorial: https://www.youtube.com/watch?v=7xHsRkOdVwo
4. Principal Component Analysis (PCA): PCA is a dimensionality reduction technique that can help you visualize high-dimensional data by projecting it onto a lower-dimensional space. It does this by finding the principal components, which are linear combinations of the original features that capture the maximum amount of variance in the data.
Principal Component Analysis (PCA) is a widely used dimensionality reduction technique that helps analyze and visualize high-dimensional data by projecting it onto a lower-dimensional space. The primary goal of PCA is to identify the directions (principal components) in which the data varies the most and project the data points onto these new axes to simplify the dataset while retaining as much information as possible.
Here’s an overview of the PCA process:
- Standardize the data: Scale the features of the dataset to have a mean of 0 and a standard deviation of 1. Standardizing is important because PCA is sensitive to the scales of the input features.
- Compute the covariance matrix: Calculate the covariance matrix for the standardized data to understand how the features are correlated with each other.
- Calculate eigenvectors and eigenvalues: Compute the eigenvectors (principal components) and corresponding eigenvalues of the covariance matrix. Eigenvectors represent the directions of the new axes, while eigenvalues signify the amount of variance explained by each principal component.
- Sort eigenvectors by eigenvalues: Arrange the eigenvectors in descending order of their corresponding eigenvalues. The eigenvector with the highest eigenvalue is the first principal component, which captures the most variance in the data.
- Select the top K eigenvectors: Choose the top K eigenvectors based on the desired dimensionality of the reduced space. These selected eigenvectors form a new matrix called the projection matrix.
- Project the data onto the new axes: Multiply the standardized data by the projection matrix to transform the original dataset into a lower-dimensional space.
PCA offers several advantages:
- It helps visualize high-dimensional data by projecting it onto a lower-dimensional space, making it easier to explore and understand the data.
- It can improve the performance of machine learning algorithms by reducing noise and computational complexity.
- It can help identify patterns and trends in the data by capturing the most significant sources of variation.
However, PCA also has some limitations:
- PCA assumes that the principal components are linear combinations of the original features, which may not hold true for all datasets.
- PCA can sometimes lead to loss of information, as it only retains the directions of maximum variance while discarding the remaining variance in the data.
- PCA is sensitive to the scales of the input features, so standardizing the data is crucial.
To gain a deeper understanding and see examples, you can check out the following resources:
- Scikit-learn’s PCA documentation: https://scikit-learn.org/stable/modules/generated/sklearn.decomposition.PCA.html
- Coursera’s “Applied Data Science with Python” course: https://www.coursera.org/specializations/data-science-python
- StatQuest’s “PCA in Python” video tutorial: https://www.youtube.com/watch?v=Lsue2gEM9D0
For more detailed explanations and examples, I recommend checking out the following resources:
- Scikit-learn’s user guide on unsupervised learning: https://scikit-learn.org/stable/unsupervised_learning.html
- Coursera’s “Machine Learning” course by Andrew Ng: https://www.coursera.org/learn/machine-learning
- DataCamp’s “Unsupervised Learning in Python” course: https://www.datacamp.com/courses/unsupervised-learning-in-python